Getting Argument Types in a Function in C++
Sometimes you want to just get the nth-argument of a function in C++, which can be less straight forward than first assumes. In this article I will explain how to get there and which steps are necessary to get this.
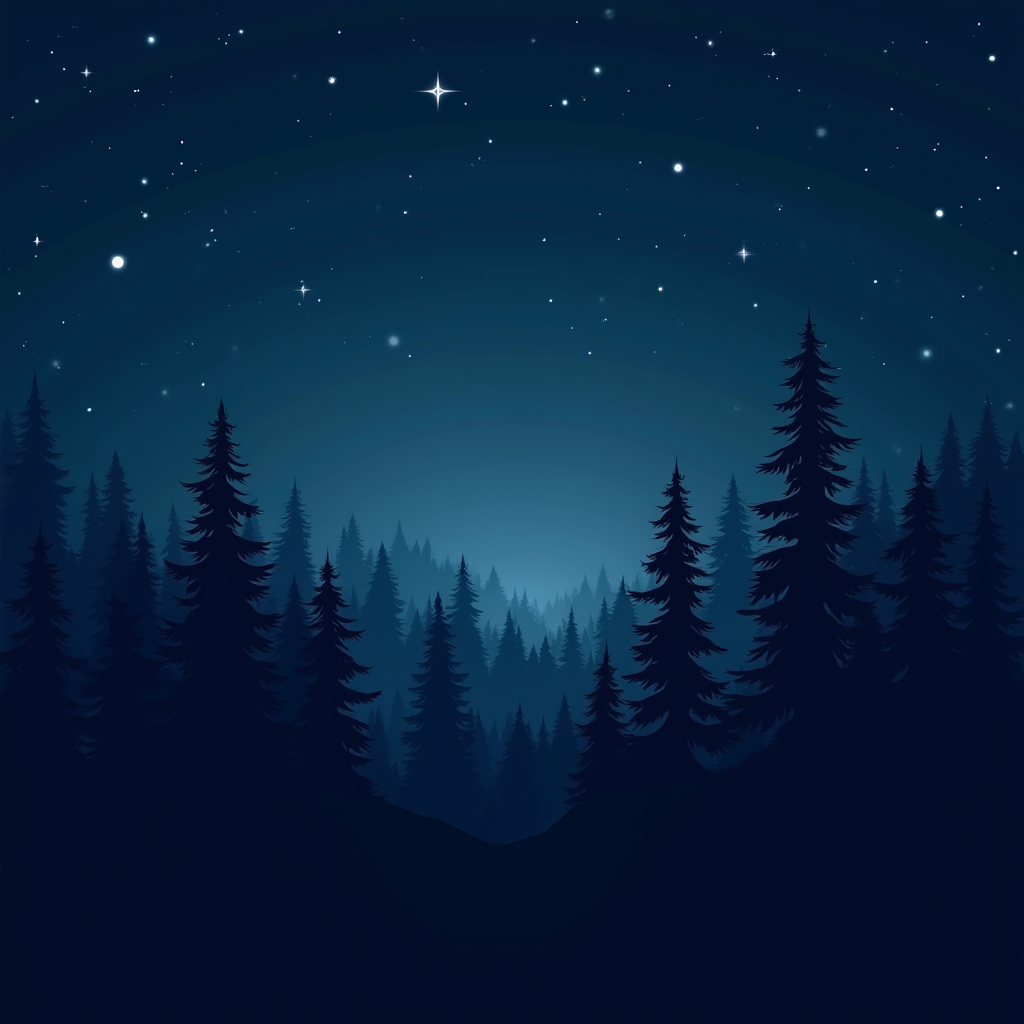
Sometimes you want to just get the nth-argument of a function in C++, which can be less straight forward than first assumes. In this article I will explain how to get there and which steps are necessary to get this.
A naive aproach would be to simply get an argument using the following template:
template <typename T>
struct FirstArgument;
template <typename ReturnType, typename Arg, typename... Args>
struct FirstArgument<ReturnType(Arg, Args...)> {
using Type = Arg;
};
template<typename T>
using FirstArgumentT = FirstArgument<T>;
When testing this it works as expected:
void f1(char a);
void f2(int a);
void f3(double a);
int main() {
std::cout << "f1: " << typeid(FirstArgumentT<decltype(f1)>).name() << '\n';
std::cout << "f2: " << typeid(FirstArgumentT<decltype(f2)>).name() << '\n';
std::cout << "f3: " << typeid(FirstArgumentT<decltype(f3)>).name() << '\n';
}
But what about getting the nth argument? Well now we need a bit of template recursion to get this done: